Podcast: Play in new window | Download
Subscribe: Apple Podcasts | Spotify | TuneIn | RSS
It’s time to get back to basics. It’s easy as a software developer to be working on the latest and greatest frameworks, using the best methodologies, trying out new things. Sometimes it’s a good idea to get a refresher on the very basic fundamentals. In this episode we go over the access modifiers that are common in C# and Java as well as ways to emulate these types of behaviors in Javascript. And let’s be honest – encapsulation is only effective if you’re providing programmers that come after you with a roadmap of how things should work. For that reason we also discuss Command Query Separation and some other ideas and practices that are a part of good programming practices. Be sure to head over to www.CodingBlocks.net/review and leave us a review on your favorite podcasting platform!
News and Updates
.NET Core CLR is now Open Source and on Github:
https://github.com/dotnet/coreclr
Want to know what Garbage Collection looks like under the covers?
https://raw.githubusercontent.com/dotnet/coreclr/master/src/gc/gc.cpp
Attended an ECMAScript 6 Meetup
Here’s a nice description of many of the features in ECMA Script 6:
https://github.com/lukehoban/es6features
Google Transpiler allowing you to write ECMAScript 6 features that will work in today’s browsers:
https://github.com/google/traceur-compiler
Extremely cool UI automated testing framework that we can’t discuss just yet! Hopefully soon…
Attended another AngularJS Meetup. Didn’t walk away with much usable information.
New Year’s Resolution Updates from The Coding Blocks Guys
Encapsulation
First, you must hear Joe’s analogy of encapsulation – if for no other reason than to hear the awkward analogy, this episode is a must listen.
The definition we came up with…
“The process of hiding internal implementation of your behavior and data, and only exposing the behaviors and properties you want people to interact with.”
Access modifiers:
- public – open to anyone, in any assembly, anywhere
- protected – any code that is in the base class where the variable is defined as protected, or is a subclass, can access the protected variables
- internal – (in Java, if you don’t put an access modifier on the variable/method, then it is essentially internal) – in C# – anyone in the assembly (dll) can access the variable. In Java, anything in the package can interact with the variable
- protected internal – (really? why would we do this to ourselves, or you) – variable can be accessed from anything within the same assembly (internal) or by anything that derives from the class in an external assembly (protected) – maybe overly complicated for most uses?
- private – cannot be seen by anything outside the class where it’s defined
C# 6 Private Protected abomination?
http://stackoverflow.com/questions/22856215/what-is-the-meaning-of-the-planned-private-protected-c-sharp-access-modifier
Global variables – good? bad? insane?
- Not reusable
- Difficult to track down errors
Encapsulation can be used as a roadmap to help programmers that follow behind you understand what they can and should use.
Seal your classes (final in Java)?
Josh Bloch – “Design for inheritance, or prohibit it.”
Friend assemblies
https://msdn.microsoft.com/en-us/library/0tke9fxk.aspx
Command Query Separation
Basically a way to make your code more “readable” or understandable by following certain conventions.
http://en.wikipedia.org/wiki/Command%E2%80%93query_separation
Commands are Mutators (they change the state of your object)
– Have return types of void
Queries are readers – don’t change the state of your object – all the do is give you back some information
– Have return types of…well, you’ll have to listen to various ways you could do this
Null checking and a number of approaches to solving this age old problem – even in an Object Oriented approach….”Maybe”
PLEASE LEAVE US A REVIEW!!!
Encapsulation in Javascript
Uses prototypical inheritance
If you want to hide variables like a “private” variable in a true OO language, you’ll need to use a closure in Javascript
IIFE’s are used to avoid creating global variables
Don’t forget to use var – if you do, bad things will happen, and you’ll have no idea why!!!
Joe said he could open up the console and view all the variables defined in the Javascript class…I don’t think he can! 🙂 I put together a JSFiddle to demonstrate encapsulation with private variables utilizing closures in Javascript:
http://jsfiddle.net/dbdbp2se/
Dependency Injection and Inversion of Control vs Encapsulation?
Need to know too much about how to instantiate your objects is against the whole idea of encapsulation. Here’s a couple of interesting reads on the topic.
http://www.daedtech.com/encapsulation-vs-inversion-of-control
Smack Dizzle: http://stackoverflow.com/questions/18547909/what-would-be-the-most-powerful-argument-for-writing-solid-applications
References
Pluralsight course: Encapsulation and SOLID
http://www.pluralsight.com/courses/encapsulation-solid
Javascript and prototypical inheritance:
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Inheritance_and_the_prototype_chain
CLR via C#
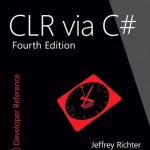
Get a Copy
http://www.amazon.com/CLR-via-Edition-Developer-Reference/dp/0735667454
Tips of the Week
Michael Outlaw’s Tip: Want to find out if you can do something in one browser or another?
http://caniuse.com/
Joe’s Tip: RogueSharp – a starting point for creating your very own Rogue-like video game using C# and .NET:
https://bitbucket.org/FaronBracy/roguesharp/
Allen’s Tip: You can’t uninstall a program in Windows because the option isn’t there? Oh…when there’s a will, there’s a way:
https://msdn.microsoft.com/en-us/library/aa372105%28v=vs.85%29.aspx