Podcast: Play in new window | Download
Subscribe: Apple Podcasts | Spotify | TuneIn | RSS
This week we’re talking about LINQ, what’s so special about .NET, the differences IQueryable and IEnumerable, and another round of “Never Have I Ever”. Oh, and jokes!
Download the episode on iTunes or Stitcher and make sure to send us your feedback!
What is LINQ?
- Language Integrated Query
- Common interface for working with data
- Famous for it’s fluint style, expressiveness, deferred execution and unconventional syntaxes
- Great for combining different data sources
“Linq enables you to write code that expresses the intent, not the mechanism.”
@Aaronaught on StackExchange.com
“Famous” LINQ Projects
What’s so special about .NET
Check out our blog post: What’s So Special About LINQ?
CLR Features
- Delegates (Func/Action/Predicate)
- Expression Trees
- Anonymous Types/Methods
- Generics
C# Features
- Lambda / Comprehension Syntax/li>
- Type inference – no string x => x.Trim()!
- Extension Methods
- Yield statement
IQueryable vs IEnumerable
- IQueryable implements IEnumerable
- IQueryable is generally “smarter” than IEnumerable
- Great post on Code Project
- Great Answer on StackOverflow
- It’s tough to talk about LINQ intelligently
Tough Questions
What exactly is deferred execution?
- Predictability vs Performance
- “Greedy” methods force enumeration
- Streaming/Sequences in C# in Depth
- Yield statement
- Multiple Enumeration Squigglies in Resharper
Syntax – Query vs Lambda
- Joins are difficult in Lambda
- From Before Select!
- You can mix syntax!
- Are Lambdas / Comprehensions truly equivalent?
LINQ Traps
- LINQ/Entity statements can provide vastly different SQL – Use SQL Profiler
- Advanced features like NOLOCKS, CTE are tough in LINQ
- Lazy rendering will bomb if ditch your context before enumerating
- Don’t forget to check for nulls in your collections!
- Date math in the LINQ statement – set before the LINQ statement
- () => {} – Isn’t “simple” the point?
- ToList() Creates Copies
Never Have I Ever…
- My lambda expressions are always just a few lines…
- My lambda expressions are never mixed with Comprehension and vice versa…
- Now that I have LINQ to SQL, I never have to write use SQL directly again…
- I’ve never written my first version as a foreach / for loop only to refactor it as a LINQ / lambda expression
- Egregious ToList() Abuse
- Used a List instead of an IEnumerable
- Multiple enumeration error
- Egregious casting
- Written out a for loop only to have Resharper complain that it could be better written as a LINQ expression
- Fallen in love with a linq method
- De-anonymize your lambdas (var x = () => Console.WriteLine())
- Cursed the lack of “WhereNot”
I’m addicted to the ForEach method. There, I said it!
Tips / Tricks
Additional Resources
- C# in Depth (author Jon Skeet is awesome btw)
- LINQ in Action
- LINQ Fundamentals on Pluralsight
- LINQ Architechture
- C# Events, Delegates, and Lambdas
- Aspectacular
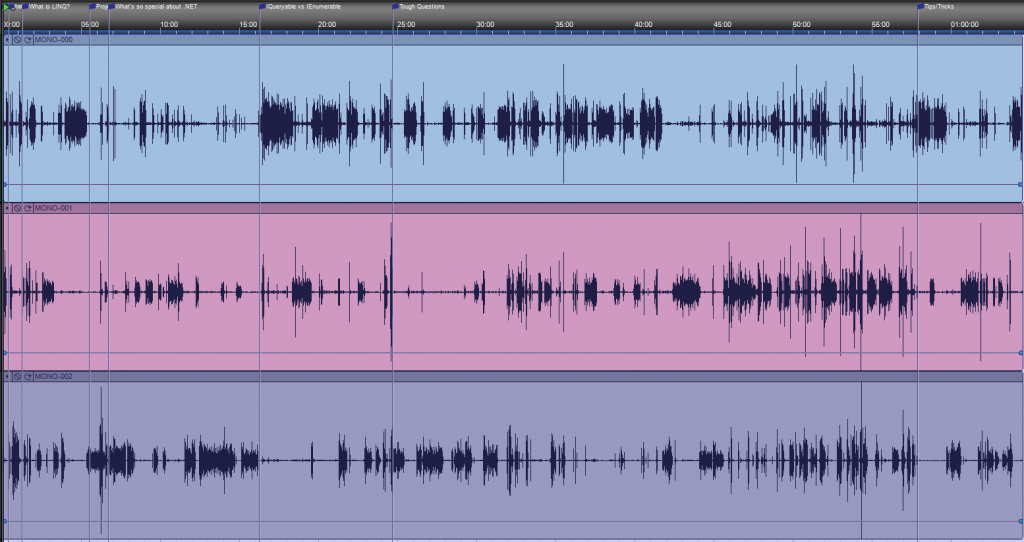